本文共 1409 字,大约阅读时间需要 4 分钟。
14黑马QT笔记之ui设计器中的常用控件01
1 先看一下各种控件整体图。
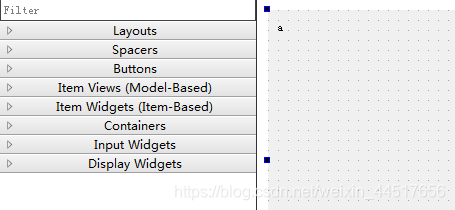
然后我们逐个说明每个分类中常用的控件。
1)第一个是对窗口布局(Layouts)用的,主要用前两个水平布局和垂直布局。
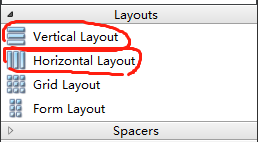
2)第二个是弹簧(Spacers),主要用于填充空白,使控件布局时能够放在想要的位置。同样也是分为水平的弹簧和垂直的弹簧。
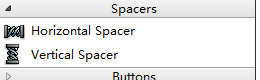
3)第三个是按钮类(Buttons)。选项1是常用的按钮;选项2是工具型按钮,与选项1区别不大;选项3是单选项按钮,只能选择点击一个;选项4则是多选项按钮,可以勾选多个;选项5是指示命令按钮,一般是指导别人如何选择的按钮;选项6是对错按钮,例如问题对话框的Yes或No按钮,具体可以根据情况选择两个按钮的内容。
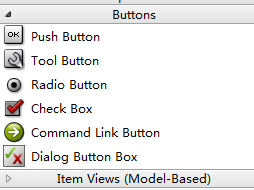
他们在窗口显示的形状:
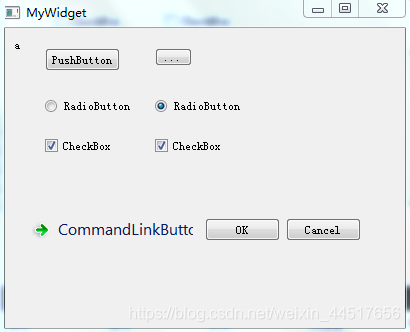
4)(4和5在数据库时再用)第四个是项目视图,主要用于显示。具体需要你用过才知道。第三个我用他显示过扇形的数据统计。(后面补)
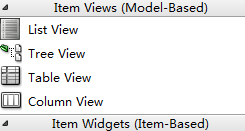
他们在主窗口主要用于某部分区域的显示,如下,从左往右,从上往下我依次显示他们,都是在某个区域显示:
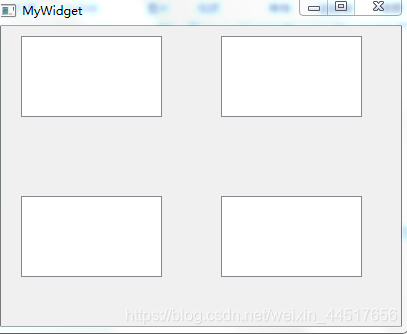
5)我记得好像是用于数据的存储,先将数据存储到这里的表,再利用4)的视图去显示。4和5作用不是很清楚,具体复习到后面再回来写。(后面补)
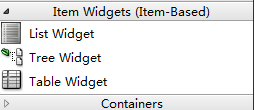
他们在窗口显示的形状:与4)差不多,只有你使用才知道区别。
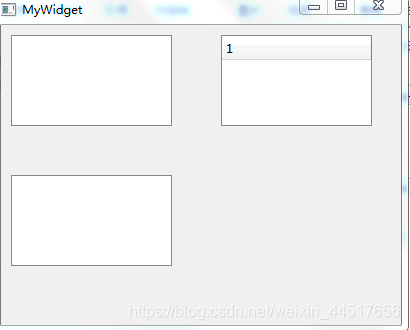
6)第六个是容器类,容器范围内可以放其他控件。选项1可以看你右下角的日期容器。选项2容器是当你界面不够大时,可以将右边的竖条往下拉(例如你浏览器的右边往下拉看其他内容)或者下方的横条往右拉,将显示不全的内容显示。选项3是抽屉式容器,他分为两页,做项目汽车管理时用到过。选项4是标签类的容器,例如notepad++打开多个文件时的显示方式。选项5是栈容器,背景是透明的,他和4也可以是多页,但不能直接点击切换页,需要额外增加按钮来调用函数切换页数,页数从下标0开始算。选项6和7基本一样,是窗口容器,6带边框,7不带,他们的作用是将一个主窗口分成多个小窗口,利用它们将小窗口的控件布局,从而达到使整个主窗口布局更美观。选项9是浮动窗口。选项8和10基本不怎么用。
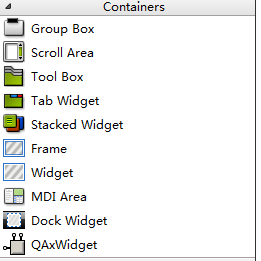
7)第七个是输入控件类。常用的是选项1是下拉框,可以添加自己的内容;选项2是字体选择下拉框,他已经提供了相应字体;选项3是行编辑,可以设置显示方式(函数为setEchoMode),例如密码方式,提示方式(QCompleter类和QStringList类用于要保存提示的字符串提供给第一个类)等等;选项4文本编辑区;选项5和选项4的区别是,4可以显示图片,5只能显示文字;选项6是调度框,一个一个增加减少的那种;选项7也是调度框,不过7是浮点型,6是整型;选项8也是调度框,只不过是用了调时间用的。选项9是调日期的调度框;选项10是日期和时间一起的调度框;选项11是某些特定情况下用到的工业元件,一般不用;选项12、13是横向和竖向滚动条,与容器的选项2类似;选项14、15是横向和竖向滑块,例如你的音量调节就是竖向滑块;最后一个一般用于快捷键的设置。
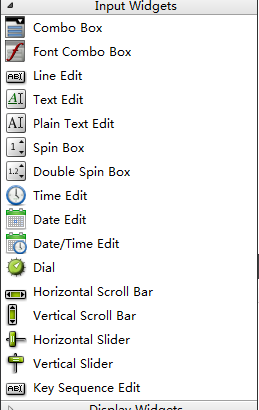
8)第八个是展示控件。常用的控件是,选项1是标签;选项4是日历,你可以在你设置的软件上放上它;选项5是LCD数码管计时器;选项6是进度条,例如你文件传输传输了多少就是使用它表示,使用最小值,当前值,最大值控制;选项7、8是水平、垂直分割线,例如之前菜单栏的菜单项就是使用水平分割线。最后两个是QML语言用到 的,我们不用。
转载地址:http://wyfv.baihongyu.com/